Creating a simple website using HTML and CSS involves writing basic code to structure and style your pages. Start with a text editor and save your file with a.html extension.
Building a simple website is an excellent way to learn web development. HTML provides the structure for your content, while CSS styles it to make it visually appealing. By combining these two languages, you can create everything from a personal blog to a professional portfolio.
This process encourages creativity and technical skills. Understanding HTML and CSS also lays the foundation for more advanced web technologies. Whether you aim to showcase your work or share your thoughts, mastering these basics will empower you to build your online presence effectively. Start with simple layouts and gradually enhance your skills as you grow.
Getting Started With Html
Creating a simple website using HTML and CSS is fun and rewarding. HTML is the backbone of any web page. It structures the content, while CSS makes it look great. Let’s dive into getting started with HTML!
Basic Structure
The basic structure of an HTML document is simple. Every HTML page begins with a html declaration. This tells the browser to expect HTML5. Then, the document is wrapped in tags.
Here’s the essential layout you’ll need:
html
Welcome to My Website!This is a simple website.
In this structure:
- : Contains meta-information about the document.
- : Sets the title shown in the browser tab.
- : Holds the content visible on the page.
Using this basic structure allows you to create a solid foundation for your website. Always remember to close your tags properly!
Essential Tags
Tags are the building blocks of HTML. Some essential tags help organize your content effectively. Here are a few important ones:
Tag | Description |
---|---|
Main heading of the page. | |
Defines a paragraph. | |
Creates an unordered list. | |
Creates an ordered list. | |
Defines a hyperlink. |
Each tag serves a unique purpose:
- to: For headings.
- : For paragraphs of text.
- : To embed images.
- : For grouping elements.
Using these essential tags makes your website more organized. They help search engines understand your content better too.
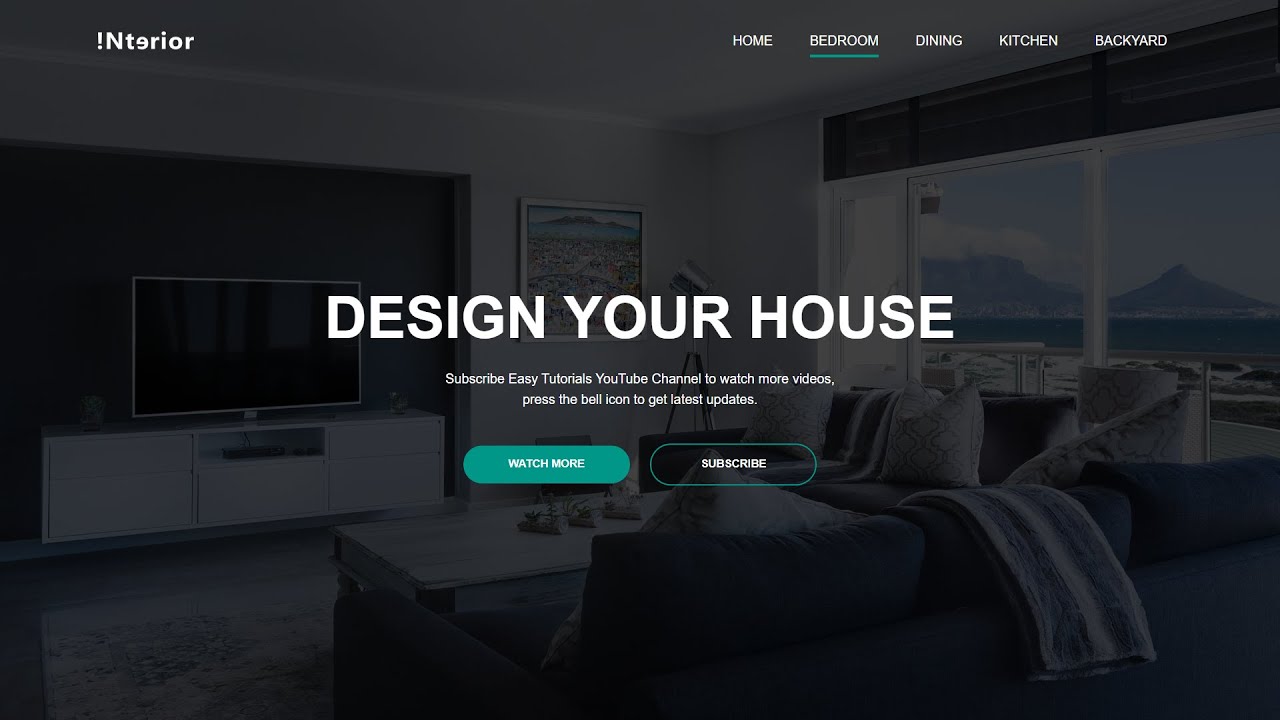
Setting Up Your Project
Creating a simple website using HTML and CSS is fun and rewarding. Setting up your project is the first step. This process involves picking the right tools and organizing your files. A good start can make your website-building journey smooth and enjoyable.
Choosing A Text Editor
Picking the right text editor is crucial for your project. A text editor helps you write and edit your HTML and CSS code. Here are some popular choices:
- Visual Studio Code: Offers many features and is free.
- Sublime Text: Fast and user-friendly.
- Notepad++: A simple editor for beginners.
Consider the following points before choosing:
- Is it easy to use?
- Does it support syntax highlighting?
- Can you customize it with plugins?
Here’s a quick comparison of the three editors:
Editor | Price | Features |
---|---|---|
Visual Studio Code | Free | Extensions, debugging, Git support |
Sublime Text | Free trial, $80 for license | Fast, distraction-free mode |
Notepad++ | Free | Lightweight, supports many languages |
Choose the one that fits your needs best. A good text editor makes coding easier and more fun!
File Organization
Good file organization helps keep your project tidy. It makes finding files easier. Start by creating a main folder for your website. Inside, add these subfolders:
- css: For your CSS files.
- images: For all image files.
- js: For JavaScript files (if needed).
Your main folder could look like this:
MyWebsite/
├── css/
├── images/
└── js/
Next, name your files clearly. Use descriptive names like:
- style.css for your main CSS file.
- index.html for your homepage.
Clear naming and organization help you find things quickly. This way, you can focus more on building your website and less on searching for files.
Building Your First Html Page
Creating a simple website using HTML and CSS is an exciting journey. Building your first HTML page can feel like magic. You create something from nothing. This guide will help you step by step. Let’s dive into how to build your first page!
Creating A Header
A header is the first thing visitors see. It sets the tone for your page. You can make a header using the
to
tags. The
tag is the biggest. It is often the title of your page. Here’s how you can create a simple header:
Welcome to My First WebsiteThis is a Subheading
Use the following tips to make your header stand out:
- Choose a catchy title.
- Keep it short and clear.
- Use keywords for SEO.
Here’s a table showing header tags:
Tag | Size | Usage |
---|---|---|
Largest | Main title | |
Medium | Subheading | |
Smaller | Section title |
Make your header attractive. Use colors and fonts with CSS. This will make your header pop!
Adding Content
Content makes your website useful. It tells visitors what your site is about. You can add text, images, and links. Use paragraphs for clear information. Here’s how you can add text:
This is my first website!I am learning HTML and CSS.
Use images to make your page lively. Images can be added using the tag:
Here are some tips for adding content:
- Keep paragraphs short.
- Use bullet points for lists.
- Include links to helpful resources.
Consider using headings for different sections. This will help organize your content:
About MeI love coding and creating websites.
With these simple steps, you can build engaging content. This will keep visitors interested in your site!
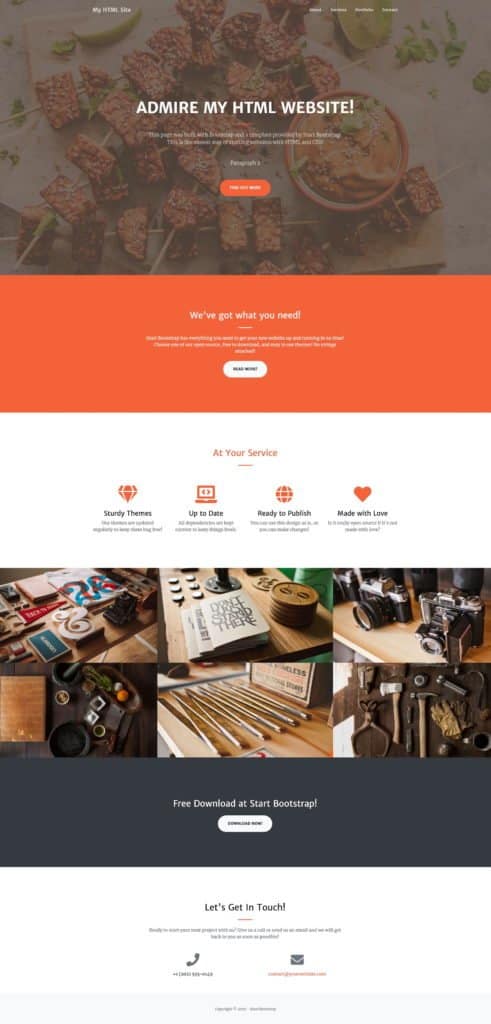
Styling With Css
Creating a simple website using HTML and CSS is fun and rewarding. Styling with CSS makes your website look beautiful and engaging. CSS controls colors, fonts, and layouts. With just a few lines of code, you can transform a plain website into a stunning one.
Selectors And Properties
CSS uses selectors to target HTML elements. Properties define how those elements will look. Here are some common selectors:
- Element Selector: Targets all instances of an element. Example:
p { color: blue; }
- Class Selector: Targets elements with a specific class. Example:
.example { font-size: 20px; }
- ID Selector: Targets a unique element. Example:
#header { background-color: gray; }
Each selector can have multiple properties. Here’s a table showing some common CSS properties:
Property | Description |
---|---|
color | Changes the text color. |
font-size | Sets the size of the text. |
background-color | Changes the background color of an element. |
margin | Creates space around elements. |
Understanding selectors and properties is key. It helps you control how your site looks. Use them wisely to create stunning designs.
Applying Styles
Applying styles in CSS is straightforward. You can write CSS in three ways: inline, internal, and external.
- Inline CSS: Use the
style
attribute within an HTML tag. Example:This text is red. - Internal CSS: Place CSS within a tag in the . Example:
- External CSS: Link a separate CSS file using the tag. Example:
Choose the method that suits your needs. External CSS keeps your HTML clean. It’s easier to manage styles in one file.
Remember to test your styles. Check how they look on different devices. This ensures a great user experience.
Creating Layouts
Creating a simple website using HTML and CSS is fun and easy. One important part of this process is creating layouts. A good layout helps visitors understand your site. It organizes content clearly. Two popular methods for designing layouts are Flexbox and the Grid System. Both tools help arrange elements on your webpage effectively.
Flexbox Basics
Flexbox stands for “Flexible Box”. It allows you to design a layout easily. With Flexbox, you can control the direction, alignment, and size of items in a container.
Here are some key properties of Flexbox:
- display: flex; – This turns the container into a flex container.
- flex-direction – This defines the direction of the items (row or column).
- justify-content – This aligns items along the main axis.
- align-items – This aligns items along the cross axis.
Here’s a simple example of Flexbox:
.container {
display: flex;
flex-direction: row;
justify-content: space-between;
align-items: center;
}
.item {
flex: 1;
margin: 5px;
}
This code creates a row of items spaced evenly. Flexbox is perfect for small layouts and navigation bars. It makes responsive design easier. Items adjust to different screen sizes automatically.
Grid System
The Grid System is another powerful layout tool. It allows you to create complex layouts with rows and columns. This system uses a two-dimensional approach.
Here are some basic features of the Grid System:
- display: grid; – This turns your container into a grid container.
- grid-template-columns – This defines the number of columns.
- grid-template-rows – This defines the number of rows.
- grid-gap – This adds space between rows and columns.
Here’s how to use the Grid System:
.container {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-gap: 10px;
}
.item {
background-color: lightblue;
padding: 20px;
}
This example creates a grid with three equal columns. Each item has space around it. The Grid System is great for larger layouts. It allows precise control over placement. You can create complex designs easily with just a few lines of code.
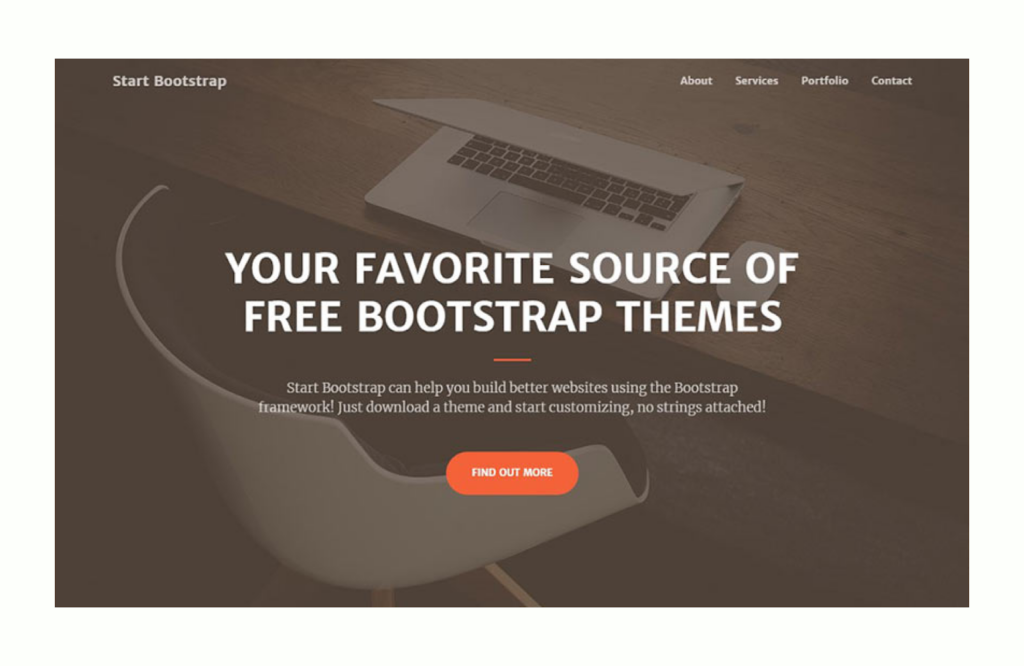
Adding Images And Media
Creating a simple website using HTML and CSS is fun and rewarding. One important part of web design is adding images and media. Images and videos make your site lively and interesting. They grab attention and keep visitors engaged. Learning how to insert them correctly is key to a great website.
Inserting Images
Images bring your website to life. They tell stories and explain ideas quickly. To add an image, use the tag. This tag requires a few attributes:
- src: This is the URL or path of the image.
- alt: This describes the image. It helps with accessibility.
- width: This sets the image width.
- height: This sets the image height.
Here is a simple example:
It’s important to choose good images. High-quality images look better and load faster. Use a variety of images to keep your content interesting. You can follow these tips:
- Use relevant images that match your content.
- Optimize image sizes to improve loading speed.
- Consider using SVG for logos and icons.
Here’s a table showing common image formats:
Format | Use Case |
---|---|
JPEG | Photos and complex images |
PNG | Images with transparency |
GIF | Simple animations |
Embedding Videos
Videos are a powerful tool for engaging your audience. They can explain complex ideas in a fun way. To embed a video, use the tag. This tag allows you to display videos from platforms like YouTube.
Here’s a basic example:
Replace VIDEO_ID with the actual ID of the video. Ensure the video is relevant to your content. Here are some tips for using videos:
- Keep videos short and to the point.
- Add a clear title and description.
- Make sure the video is mobile-friendly.
Remember to check the video permissions. Some videos may not allow embedding. Always respect copyright rules. Well-placed videos can enhance user experience and keep visitors on your site longer.
Final Touches
Creating a simple website using HTML and CSS is an exciting journey. The final touches are crucial in making your site user-friendly and visually appealing. This stage focuses on ensuring your site looks great on all devices and performs well. Let’s dive into the essential final steps to enhance your website.
Responsive Design
Responsive design ensures your website looks good on any device. This includes desktops, tablets, and smartphones. A responsive site adapts its layout and content to fit different screen sizes. Here are some key practices for achieving responsive design:
- Use Flexible Grids: Create a grid system that adjusts based on the screen size.
- Set Viewport: Use the viewport meta tag to control layout on mobile browsers.
- Media Queries: Implement CSS media queries to apply different styles based on the device.
Here’s a simple example of a media query:
@media (max-width: 600px) {
body {
background-color: lightblue;
}
}
This code changes the background color on screens smaller than 600 pixels. Test your site on various devices to see how it looks. Tools like Chrome DevTools allow you to simulate different devices easily.
Remember, a responsive website improves user experience. It can also help with SEO. Search engines prefer sites that are mobile-friendly.
Testing Your Site
Testing your site is vital. It ensures everything works as intended. Start by checking all links. Make sure they lead to the correct pages. Here are some key areas to focus on:
- Cross-Browser Compatibility: Test your site on different browsers like Chrome, Firefox, and Safari.
- Loading Speed: Use tools like Google PageSpeed Insights to check your site’s speed.
- Functionality: Ensure forms, buttons, and interactive elements work correctly.
Consider creating a checklist for your testing:
Testing Area | Status |
---|---|
Links | ✅ |
Images | ✅ |
Mobile View | ✅ |
Loading Speed | ✅ |
After testing, gather feedback from friends or family. They can spot issues you might have missed. Take their suggestions seriously. This step helps improve your site significantly.
Conclusion
Building a simple website with HTML and CSS is an achievable goal. This foundational skill opens the door to endless possibilities. With practice, anyone can enhance their web development abilities. Start creating and experimenting today. Your journey into the world of web design begins here, so embrace the learning process and enjoy the creativity.